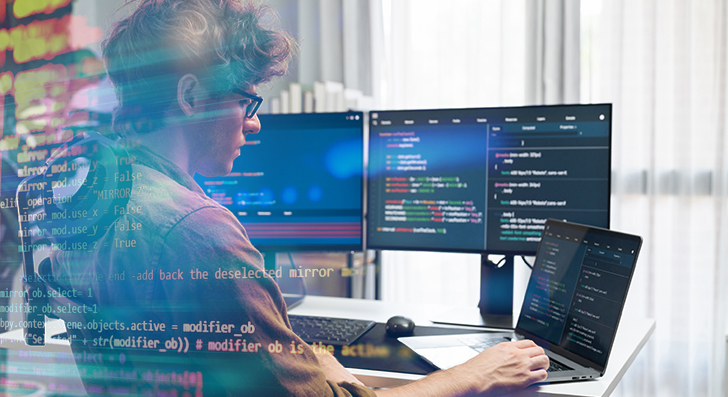
Scalability signifies your software can cope with progress—a lot more customers, extra facts, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they expand speedy since the first design and style can’t tackle the extra load. Being a developer, you need to Consider early about how your process will behave under pressure.
Start off by building your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly linked. Instead, use modular layout or microservices. These styles crack your app into more compact, unbiased parts. Each and every module or assistance can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from working day 1. Will it want to manage a million consumers or maybe 100? Pick the ideal type—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath recent conditions. Think about what would occur In case your user base doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style designs that support scaling, like information queues or party-pushed units. These assistance your application tackle extra requests without the need of having overloaded.
Once you Construct with scalability in mind, you're not just making ready for fulfillment—you might be lessening future head aches. A effectively-planned process is simpler to keep up, adapt, and expand. It’s far better to organize early than to rebuild later.
Use the Right Databases
Selecting the correct database is really a vital Component of constructing scalable programs. Not all databases are built the same, and utilizing the Improper one can slow you down and even trigger failures as your application grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational databases like PostgreSQL or MySQL is an efficient match. These are solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to manage a lot more traffic and knowledge.
If your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more simply.
Also, consider your go through and generate patterns. Do you think you're carrying out numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can manage significant compose throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Assume in advance. You might not need State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your information according to your accessibility designs. And constantly keep an eye on databases functionality while you improve.
Briefly, the appropriate databases depends on your application’s composition, velocity desires, And just how you be expecting it to improve. Just take time to choose properly—it’ll conserve lots of difficulty later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly penned code or unoptimized queries can decelerate functionality and overload your process. That’s why it’s essential to Create effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most complex Alternative if an easy 1 works. Maintain your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These normally slow matters down much more than the code by itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially throughout big tables.
When you notice precisely the same details getting asked for many times, use caching. Shop the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application more effective.
Remember to examination with substantial datasets. Code and queries that work good with 100 information may possibly crash if they have to take care of one million.
In short, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of a lot more consumers and even more targeted traffic. If almost everything goes by just one server, it can promptly become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the do the job, the load balancer routes buyers to unique servers determined by availability. This implies no single server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it from the databases every time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app extra productive.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more people, stay quickly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need instruments that permit your application grow conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase hardware or guess potential potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to target constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to independent aspects of your app into services. You may update or scale elements independently, which is perfect for performance and trustworthiness.
In brief, working with cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you want your application to expand without the need of limitations, start out utilizing these equipment early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when points go wrong. Checking allows you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you obtain and visualize this data.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even more info little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal resources, you could Construct applications that grow easily devoid of breaking under pressure. Start off compact, Believe major, and build wise.